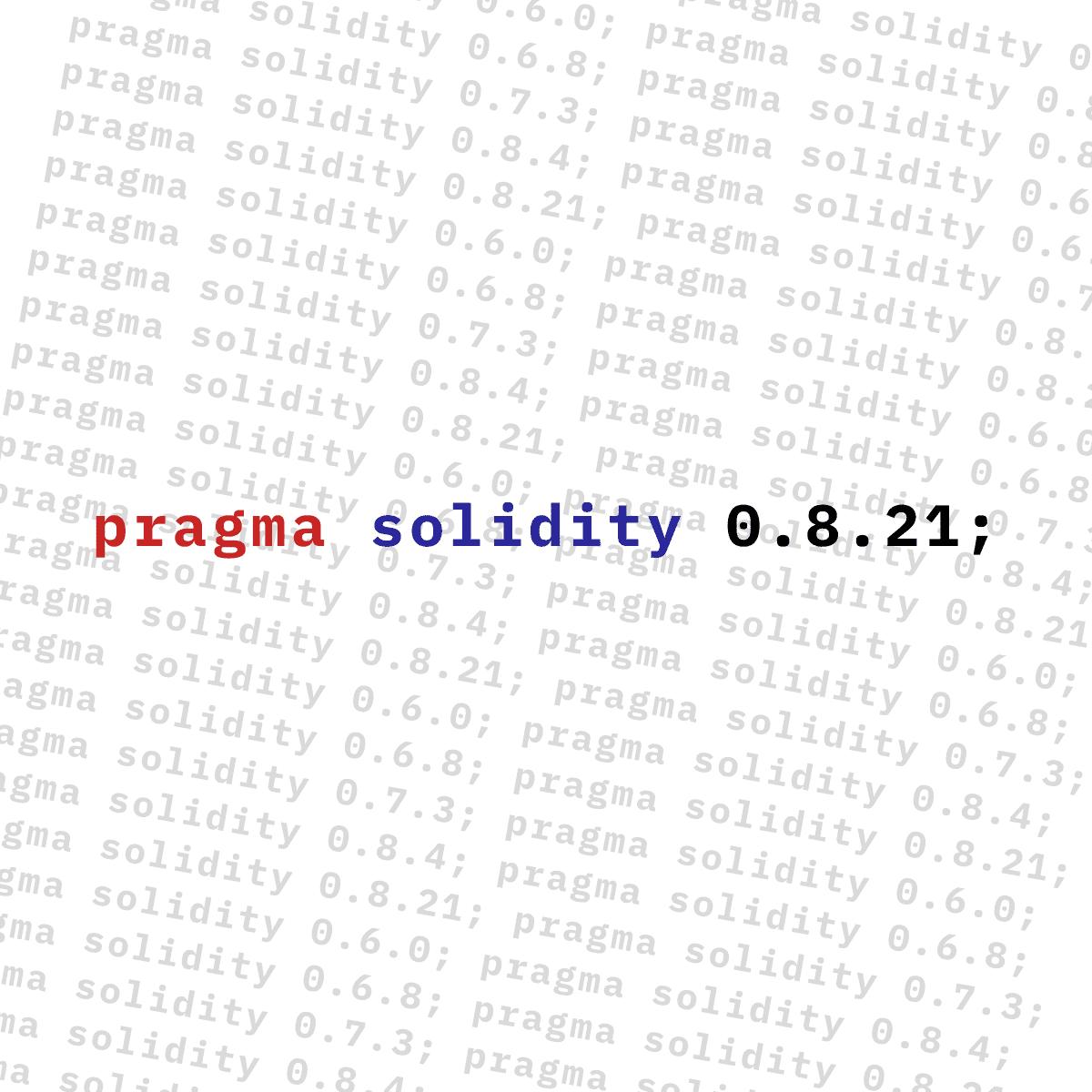
Decoding pragma in Solidity: Why It's Essential
Hey there! If you’ve ever peeked into a Solidity file, you’ve likely come across the term pragma
sitting right at the top. It might seem like a fancy term, but it’s fundamental to ensuring our smart contracts run smoothly. Let’s dive in and demystify this essential directive.
What Exactly is pragma?
In Solidity, pragma
is a directive used to specify the version of the compiler your code should be compiled with. Given how rapidly Solidity evolves, this little directive ensures that your contract is compiled with a compatible version, preventing potential bugs and vulnerabilities. As of now, the latest version in the 0.8
series is 0.8.21
.
Here’s a simple example:
pragma solidity ^0.8.21;
This tells the compiler that the code should be compiled with version 0.8.21
or any newer version until (but not including) 0.9.0
.
Why is pragma So Important?
Imagine writing a piece of code today, and then, a year later, trying to compile it with a completely revamped compiler. Sounds like a recipe for disaster, right? That’s where pragma
comes to the rescue.
- Compatibility: Solidity is continuously evolving. New versions might introduce changes that could break older contracts. By specifying a version using pragma, you ensure your contract remains compatible.
- Security: Some versions of Solidity might have vulnerabilities. By explicitly stating the version, you can ensure you’re using a secure, patched version.
- Clarity: For anyone reading your code, the pragma directive immediately clarifies which version of Solidity you’re using. It sets the stage for the rest of your code.
🤔 Did you know? The word “pragma” is derived from “pragmatic,” indicating its practical application in guiding the compiler on how to treat the source code.
Using pragma Effectively
While it’s tempting to always use the latest version, it’s essential to be cautious. Here’s a quick guide:
- Stay Updated, But Cautiously: Always be aware of the latest Solidity versions and their changes. However, don’t rush to adopt a new version without thoroughly testing it.
- Be Explicit: Instead of using
^0.8.21
, which allows any version until0.9.0
, you might want to specifypragma solidity 0.8.21;
if you want to stick strictly to that version. - Research Known Issues: Before settling on a version, do a quick check on any known issues or vulnerabilities associated with it.
How to use it?
- Exact Version: If you want your code to be compiled with an exact version of the Solidity compiler, you simply state that version. solidity
pragma solidity 0.8.21;
This tells the compiler to use version 0.8.21
and no other.
- Version Range: You can specify a range of versions suitable for your code. solidity
pragma solidity >=0.8.0 <0.9.0;
This means the code is compatible with any compiler version from 0.8.0
up to, but not including, 0.9.0
.
- Caret Versioning:
This is a common way to specify version in Solidity. The caret
^
symbol allows for updates that do not include changes that break what you’ve written. solidity
pragma solidity ^0.8.21;
This is similar to the version range example above. It implies any version from 0.8.21
up to, but not including, 0.9.0
.
🤔 Did you know? Caret versioning is borrowed from NPM’s versioning system. It’s a way to accept non-breaking updates without manually changing the version number.
- Wildcard: If you’re feeling adventurous (though it’s not recommended for production code), you can use a wildcard to specify that your code can be compiled with any version of a compiler within a certain range.
pragma solidity 0.8.*;
This means the code can be compiled with any version in the 0.8 series.
When choosing a version, always remember the importance of testing. Different compiler versions might introduce subtle changes, so always ensure your contract behaves as expected, regardless of the version you choose.
In conclusion, while pragma might seem like a small detail, it plays a pivotal role in the world of smart contracts. It’s one of those things that, when used correctly, you won’t notice—but if misused, it can lead to a world of pain. As we continue our journey into the depths of Solidity, always remember the importance of the foundation, and pragma is a significant part of that base. Stay curious, and let’s keep exploring together!