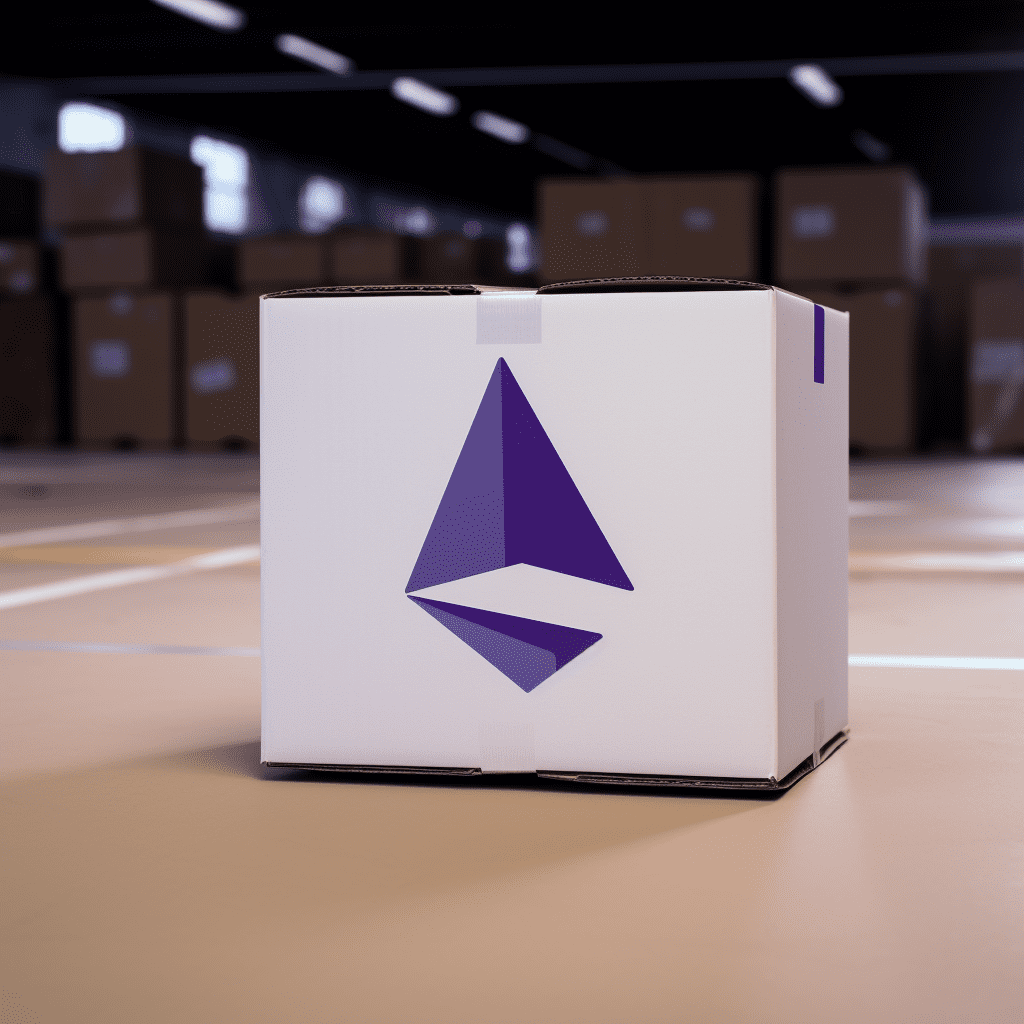
Solidity 101: Crafting & Deploying Smart Contracts Safely
Hey there! Remember when we embarked on our journey into the world of Solidity? Last time, we set up our very own development environment, and trust me, it felt like setting up base camp before a mountain climb. We dived deep into the essence of Solidity, explored the transformative power of Ethereum, and got our hands dirty by setting up the tools of the trade.
If you missed it, think of it as our prep work before the big adventure. And now? It’s time to take the next step. Let’s dive into the fascinating world of smart contracts and see how we can craft our very own digital agreements. Ready to roll? Let’s get coding!
Introduction to Solidity
Smart contracts. Even after all my time in the tech world, I’m still amazed by the sheer brilliance of this concept. But let’s break it down for those who might be hearing about it for the first time.
At their core, smart contracts are like the contracts we’re all familiar with, but they’ve been given a digital upgrade. Instead of being penned on paper, they’re coded and live on the blockchain. Think of them as a set of rules: once these rules are established, they’re set in stone, ensuring every participant plays fair.
What truly fascinates me is the trust factor. With smart contracts, the need for a middleman, like a bank, is eliminated. Everything is transparent, and the blockchain ensures that the terms are met. It’s a testament to how technology can simplify complex processes.
🤔 The first real-world transaction using Bitcoin was to buy two pizzas in 2010. Those pizzas cost 10,000 Bitcoins, which would be worth millions today! It’s a fun reminder of how far we’ve come in the world of blockchain and cryptocurrency. 🍕
As we journey further into Solidity, it’s essential to grasp the importance of smart contracts. They’re not just a trend; they’re reshaping the digital landscape, and I’m still learning every day from their vast potential.
Basics of Writing a Smart Contract
Alright, now that we’ve got a grasp on what smart contracts are, let’s roll up our sleeves and get into the nitty-gritty of writing one. Trust me, it’s not as daunting as it might seem!
First things first, every smart contract in Solidity starts with a version pragma. It’s a way to tell the compiler which version of Solidity you’re using. It looks something like this:
pragma solidity ^0.8.0;
This ensures that your contract is compiled with the right version, avoiding any unexpected behaviors.
Next, you’ll define the contract using the contract keyword, followed by its name. Inside the contract, you can declare variables, functions, and events. Variables store data, functions define the logic, and events help with logging activities.
contract MyContract {
uint256 private data; // A private state variable
event DataChanged(uint256 newData); // An event
function setData(uint256 _data) public { // A public function
data = _data;
emit DataChanged(data);
}
}
Data Types in Solidity
Solidity, like many programming languages, offers a variety of data types. Understanding these is crucial when defining the structure and behavior of your smart contracts.
- Integers: These are whole numbers which can be both positive and negative. uint stands for unsigned integer, which means it can only represent non-negative numbers. The number after uint (e.g., uint256) represents the number of bits the integer takes up, with uint256 being the most commonly used due to gas optimization.
- Boolean: Represents a true or false value.
- Address: This type holds a 20-byte value, which is the size of an Ethereum address. It’s used to represent the address of an external account or another smart contract.
- Bytes: Fixed-size byte arrays. bytes1, bytes2, bytes3, … , bytes32 are used to represent a sequence of bytes from 1 to 32.
- Strings: Represents a sequence of characters. Unlike bytes, strings can be of any length.
- Arrays: A collection of elements, which can be of any type, including another array.
- Structs: Custom defined types that can group several variables.
- Enums: A way to define user-defined types with a limited number of values.
// int / uint
uint256 positiveNumber = 123;
int256 anyNumber = -123;
// boolean
bool isTrue = true;
// address
address walletAddress = 0xdac17f958d2ee523a2206206994597c13d831ec7;
// bytes
bytes32 hashValue = "Hello World!";
// strings
string greeting = "Hello, Solidity!";
// arrays
uint256[] public numbers = [1, 2, 3];
string[5] public names; // An array that can hold 5 strings
// struct
struct Person {
string name;
uint256 age;
bool isStudent;
}
Person public student = Person("Alice", 20, true);
// enum
enum State { Created, Locked, Inactive }
State public currentState = State.Created;
🤔 Remember, choosing the right data type is essential for both representing data and optimizing gas usage.
Function Visibility
When writing functions, it’s essential to specify their visibility and whether they can modify the contract’s state. These specifications ensure your contract runs smoothly and securely.
In Solidity, functions can have different visibility levels:
- Public: Functions can be called both internally and externally.
- Private: Functions can only be called from within the contract they are defined in.
- Internal: Functions can be called from the contract they are defined in and from derived contracts.
- External: Functions can only be called from outside the contract.
// public
function publicFunction() public returns (uint256) {
return data;
}
// private
function privateFunction() private returns (uint256) {
return data * 2;
}
// internal
function internalFunction() internal returns (uint256) {
return data + 5;
}
// external
function externalFunction(uint256 _value) external returns (uint256) {
return _value * 3;
}
While Solidity might have its unique quirks, if you’ve dabbled in JavaScript (TypeScript) or other C-like languages, you’ll find many familiar elements.
Simple Counter Smart Contract Example
To help solidify our understanding, let’s dive into a basic example:
// Specifies the version of Solidity compiler this code should use
pragma solidity ^0.8.0;
// Defines our contract named "Counter"
contract Counter {
// Declares a state variable 'count' of type unsigned integer
uint256 private count = 0;
// Event that will emit the current count value
event NewCount(uint256 count);
// Function to increase the count by 1
function increment() public {
count += 1;
emit NewCount(count); // Emitting an event with the new count
}
// Function to decrease the count by 1
function decrement() public {
count -= 1;
emit NewCount(count); // Emitting an event with the new count
}
// Function to get the current count
function getCount() public view returns (uint256) {
return count;
}
}
This simple contract showcases the structure of a Solidity contract, how state variables are used, and how functions can interact with these variables. It’s a great starting point for anyone looking to get their feet wet in smart contract development!
Wrapping Up This Chapter
We’ve scratched the surface of Solidity, diving into its core components and understanding the basics of crafting a smart contract. But, as with any language, there’s so much more beneath the surface. I’ve been through the trenches, and I can tell you that Solidity has its fair share of nuances and intricacies. While we didn’t have the time to delve into every nook and cranny in this article, don’t fret! In our upcoming articles, we’ll dive deeper, exploring the more advanced aspects and peculiarities of the language.
Deploying Your First Smart Contract: Testnet First!
After getting our feet wet with the basics of Solidity, it’s time to take the next big step: deploying our smart contract onto the Ethereum blockchain. It’s one thing to write a contract, but bringing it to life on the blockchain? That’s where the real magic happens!
Embarking on the journey of deploying a smart contract can be both exhilarating and nerve-wracking. While the thrill of seeing your code come alive on the blockchain is unmatched, the process can be a bit daunting, especially with real money at stake. That’s why, before we even think of the mainnet, we’ll focus on the testnet. Trust me, it’s a lifesaver!
Why Testnet?
The testnet is a simulation of the Ethereum network. It behaves just like the mainnet, but with one crucial difference: the Ether here isn’t real. This means you can test, break things, and learn without burning a hole in your pocket. And given how deploying on the mainnet requires real money, which you can’t get back if something goes wrong, the testnet is your best friend.
🤔 Did you know? A faucet is a service that dispenses free test Ether for the testnet. It’s like a tap that drips Ether, allowing developers to test without any financial implications.
What Does “Deploying” Mean?
In the simplest terms, deploying a smart contract means taking the code you’ve written and broadcasting it to the Ethereum network. Once it’s on the network, it gets its own unique address, and voilà, it’s alive and kicking! It’s like launching a website, but instead of servers, we’re using the decentralized Ethereum network.
Tools of the Trade
Before we dive into the deployment process, let’s get familiar with some essential tools:
- MetaMask: A browser extension that acts as a bridge between your browser and the Ethereum blockchain. It’ll be your go-to for deploying and interacting with your contract. Also, it not only connects you to the mainnet but also various testnets, allowing you to test without spending real Ether.
- Remix: A powerful platform for smart contract development. It’s here that you’ll be deploying your contract to the testnet.
🤔 Did you know? MetaMask not only allows you to interact with the Ethereum mainnet but also with various testnets. This is super handy when you want to test your contracts without spending real Ether.
Deploying with Remix and MetaMask
- Setting Up MetaMask: Install MetaMask if you haven’t. Create an account and, importantly, switch to a testnet like Ropsten.
- Getting Test Ether: Since the Ether on testnet isn’t real, you can get it for free from a faucet. Just search for “Goerli faucet” or whichever testnet you’re on, enter your address, and receive your test Ether.
- Uploading Your Contract to Remix: Navigate to Remix and paste your smart contract code. Ensure it compiles without errors.
- Connecting Remix to MetaMask: In Remix, select the “Deploy & Run Transactions” tab. For “Environment”, choose “Injected Web3”. This links Remix to MetaMask.
- Deploying the Contract: With the correct contract selected, hit “Deploy”. MetaMask will prompt you to confirm the transaction. Remember, this is just test Ether, so go ahead and confirm!
- Interacting with Your Contract: Your contract will now be under “Deployed Contracts” in Remix. Here, you can play around with its functions.
A Word of Caution… Again!
I can’t stress this enough: deploying on the mainnet is expensive. It consumes real Ether, and if you make a mistake, there’s no undo button. Always, always test on a testnet first. Once you’re confident, only then consider the mainnet. And even then, proceed with caution.
And there we have it! It’s a safe, risk-free way to get your feet wet. In our next articles, we’ll delve deeper into the nuances of Ethereum and Solidity. But for now, happy testing, and remember: the testnet is your playground. Enjoy it!